Getting Started with the Indico API
Introduction
Indico provides libraries for developers to build with the IPA Platform in several popular languages. Visit any of the links below for language-specific documentation.
Register an Account
The first step to begin developing with the Indico API is to register an account on your organization's Indico platform.
Remember, you may need someone with Indico admin-access to grant you "app access" after registering to begin using the platform.
Using the Client Libraries
Obtaining an API Token
Download a token from your account page by clicking the large, blue “Download New API Token” button.
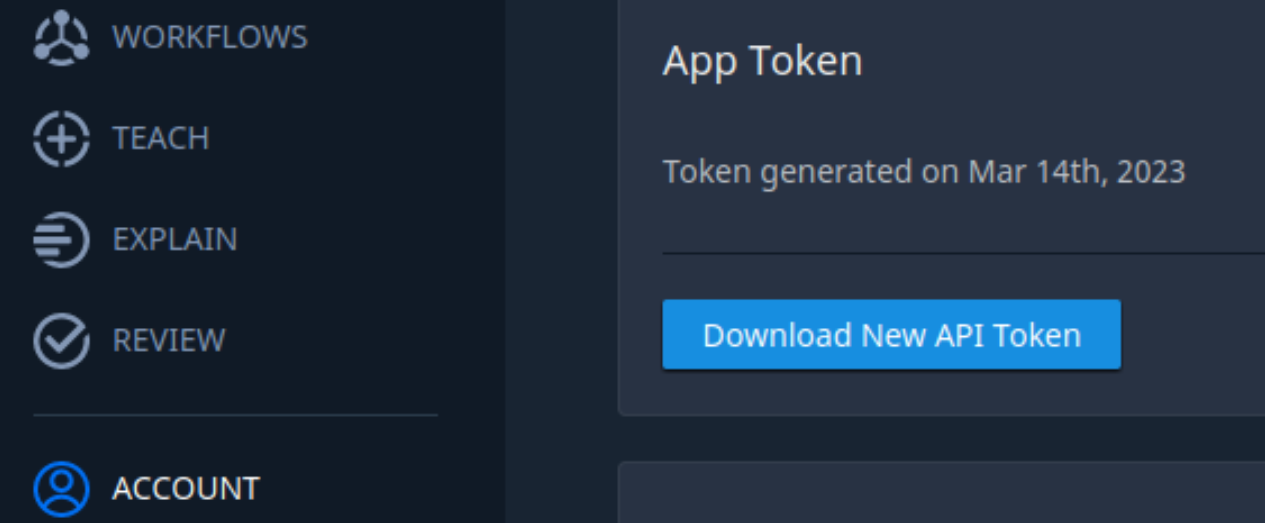
Most browsers will download the API token as indico_api_token.txt
and place it in your Downloads directory. We suggest moving the token file from Downloads to either your home directory or another location in your development environment.
Using your API Token
Indico offers three client libraries that allow developers to interact with our API using tokens.
These libraries contain pre-built classes to handle the majority of common Indico interactions. You can also send custom GraphQL requests from the libraries.
See below for example of how to use your API token to authenticate with the Indico client libraries.
from indico import IndicoClient, IndicoConfig
api_token_path = "./indico_api_token.txt"
# Create an Indico API client
my_config = IndicoConfig(
host="your-cluster.example.com", api_token_path=api_token_path
)
client = IndicoClient(config=my_config)
using System;
using IndicoV2;
namespace Examples
{
public class TokenExample
{
private static string GetToken() =>
File.ReadAllText(Path.Combine(Directory.GetCurrentDirectory(), "\\indico_api_token.txt"););
private static Uri url = new Uri("https://your-cluster.example.com")
public static async Task Main()
{
var client = new IndicoClient(GetToken(), url));
}
}
}
package com.testco.indico;
public class main {
public static IndicoKtorClient client;
private static String token_path = "./indico_api_token.txt";
private static String host = "https://your-cluster.example.com"
public static void main(String args[]) throws Exception {
/**
* Set up client
*/
IndicoConfig config = new IndicoConfig.Builder().host(host)
.protocol("https")
.tokenPath(token_path)
.build();
client = new IndicoKtorClient(config);
}
GraphQL API
All Indico accounts also have the ability to interact via our GraphQL API with models/resources that they created/uploaded or have been shared at the analyst/manager permission level.
If you have been granted GraphQL access by your administrator, you will also be able to use our web sandbox to view the full schema of available data points and test custom queries.
This page can be reached by selecting GraphiQL
in the left hand rail or adding /graph/api/graphql
to the end of your Indico platform URL.
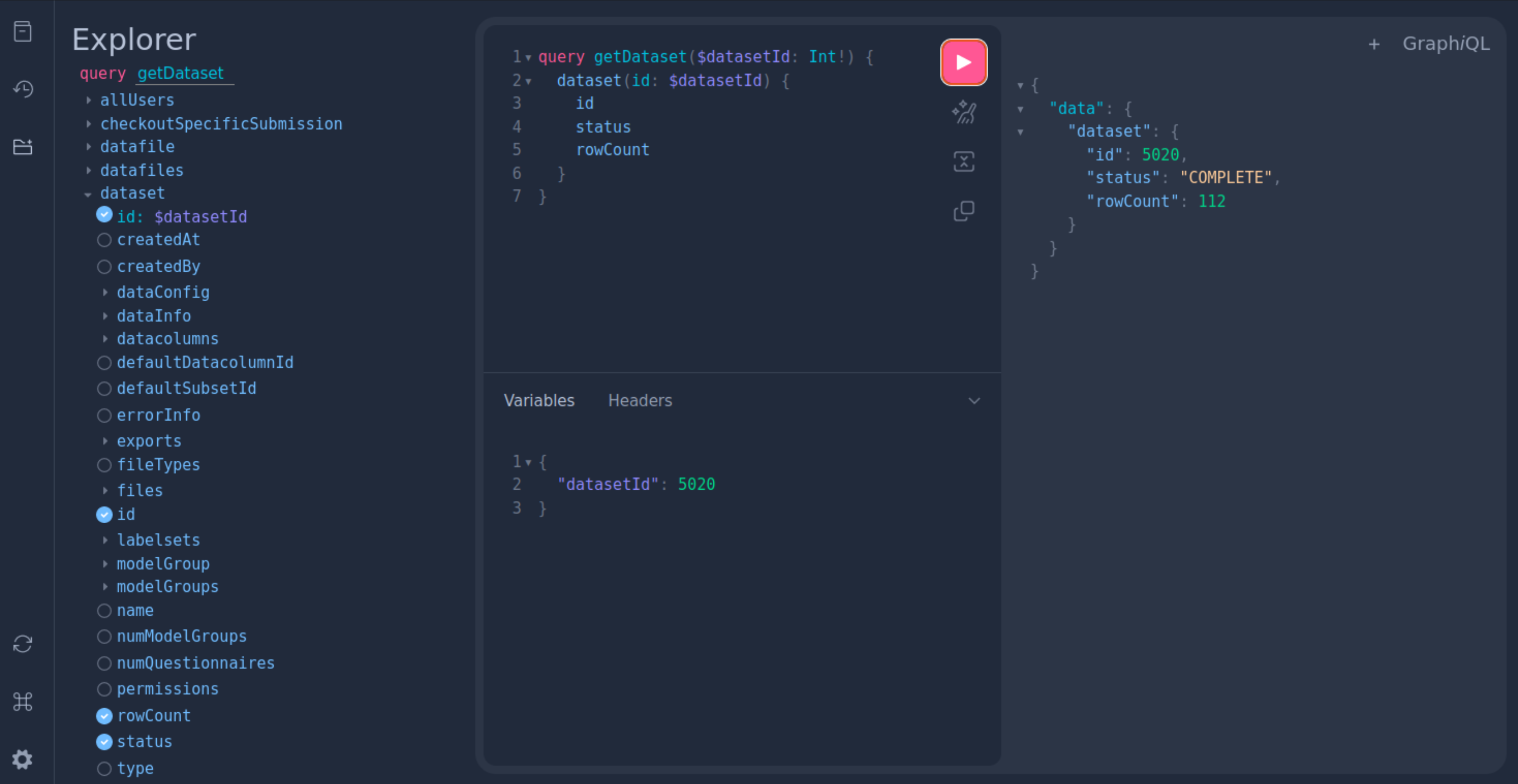
Updated about 2 months ago