Custom GraphQL Queries
from indico import IndicoClient, IndicoConfig
from indico.queries import GraphQLRequest
HOST = 'mycluster.indico.io'
TOKEN= "./indico_api_token.txt"
my_config = IndicoConfig(
host=HOST, api_token_path=TOKEN
)
client = IndicoClient(config=my_config)
QUERY = """
query MyCustomQuery($id: Int) {
dataset(id: $id) {
id
labelsets {
name
}
fileTypes
}
}
"""
variables= {
"id": 215
}
request = GraphQLRequest(query=QUERY, variables=variables)
response = client.call(request)
print(response)
You can use the GraphiQL explorer to help build custom queries and mutations.
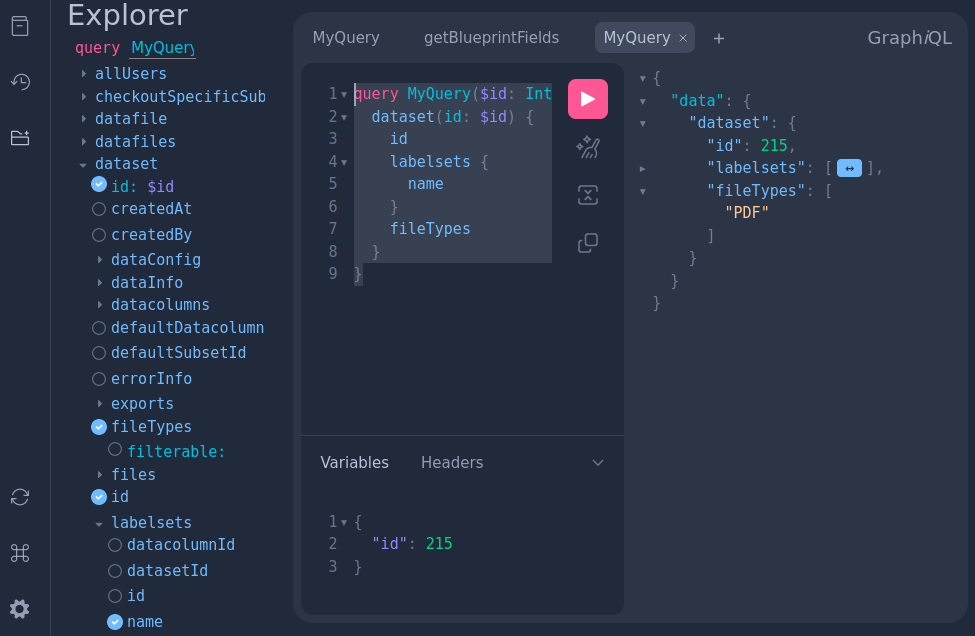
Updated 3 months ago