Exchange Integration
To submit emails to a workflow, set up an integration with a Microsoft Outlook or Exchange server as described in this guide.
Adding an Integration
Integrations can be added to the input node of any workflow based on a text or document dataset.
mutation addExchangeIntegration($workflowId: Int!,
$config: ExchangeIntegrationConfigurationInput!,
$credentials: ExchangeIntegrationCredentialsInput!)
{
addExchangeIntegrationToWorkflow(workflowId: $workflowId, config: $config, credentials: $credentials)
{
integration
{
id
enabled # will be FALSE since integrations are turned off when first added
}
}
}
Configuration
ExchangeIntegrationConfigurationInput
is a dict that contains user_id
, folder_id
, and (optionally) filters
.
{
"user_id": "jdlamvpaolsdkls", #id of the user object in Microsoft
"folder_id": "AAAnjfklalcvaldks===", #120 character folder id from Microsoft
"filters": "[{\"name\":\"All Unread Emails\"}]" #JSON string, OPTIONAL
}
User ID
The user_id
can be obtained in Azure Active Directory by clicking on the name of the user associated with the mail folder. The user_id
is the object ID for this user.
Folder ID
To obtain the folder ID, call the Microsoft GET mailFolders
API endpoint with the user ID and select the appropriate folder ID. For more information, read this Microsoft article on GET mailFolders.
Filters
So far, the available filters for which emails to process are:
- All Emails
- All Unread Emails
- All Read Emails
If no filter is specified, the default is All Unread Emails.
Credentials
ExchangeIntegrationCredentialsInput
is a dict that contains tenant_id
, client_id
, and client_secret
.
{
"tenant_id": "here's a valid tenant ID",
"client_id": "here's a valid client ID",
"client_secret": "shhh it's a secret"
}
To authenticate with Microsoft, Indico’s integration service uses the OAuth 2.0 client credentials flow described here: Microsoft identity platform and the OAuth 2.0 client credentials flow.
To obtain credentials for this flow, create a new app registration in Azure Active Directory (AD) and provide the client ID, tenant ID, and client secret.
The app needs to have the User.Read.All and Mail.Read application permissions.
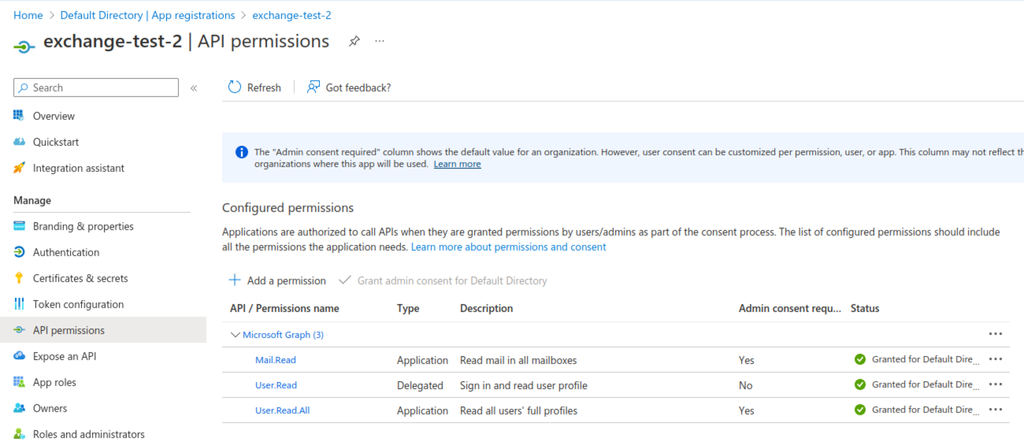
Starting an Integration
Integrations can only be started if the workflow is in a runnable state. If the specified integration is already running, this mutation has no effect.
mutation StartIntegration($integrationId: Int!){
startWorkflowIntegration(integrationId: $integrationId){
success
}
}
Pausing an Integration
If the specified integration isn’t running, this mutation has no effect.
mutation PauseIntegration($integrationId: Int!){
pauseWorkflowIntegration(integrationId: $integrationId){
success # bool
}
}
Deleting an Integration
mutation DeleteWorkflowIntegration($integrationId: Int!) {
deleteWorkflowIntegration(integrationId: $integrationId) {
success
}
}
Retrieving Submissions
When submissions are created based on emails from Exchange, the internet message ID of the email is included in the name of the submission file.
To retrieve the submission result file for a particular email:
- Obtain the email’s
internetMessageId
from Exchange. Note that theinternetMessageId
is different from the ID. For more information, read this Microsoft documentation topic. The internet message ID can be obtained using the list messages or get message Graph APIs. - Filter submission results for input files whose name includes the
internetMessageId
.
query getSubmissionsList($workflowId: Int!, $filters: SubmissionFilter) {
submissions(workflowIds: [$workflowId], filters: $filters, skip: 0, limit: 10) {
submissions {
id
createdAt
inputFilename
}
}
}
{
"workflowId": <WORKFLOW ID>,
"filters": {
"inputFilename": <INTERNET MESSAGE ID>
}
}
Updated 3 months ago